This is the fourth of a series about Application Insights and Log analytics. I’ve shown you how to add existing logs, using the log analytics query language to view you logs and how to enhance your query to drill down and get to the logs you are interested in. This post is about how you can add logs from your code and provide the information to allow you refine your queries and help you to diagnose your faults more easily
If you don’t already have application insights then you can create a new instance in the Azure portal (https://portal.azure.com/)
Get your application insights key from the azure portal. Click on your application insights instance and navigate to the Overview section then copy your instrumentation key. You will need this in your code.
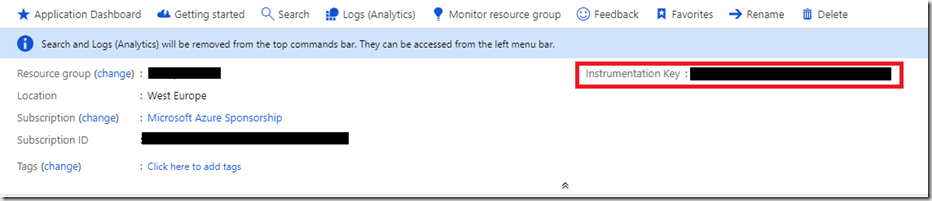
In your project, add application insights via nuget :
Install-Package Microsoft.ApplicationInsights -Version 2.10.0
In you code you need to assign the key to Application Insights as follows:
TelemetryConfiguration configuration = TelemetryConfiguration.CreateDefault();
configuration.InstrumentationKey = “put your key here”;
To log details using application insights then you need a telemetry client.
TelemetryClient telemetry = new TelemetryClient(configuration);
The telemetry client has a larger number of features than I am not going to talk about here as I am just interested in logging today. There are three methods of interest: TrackEvent, TrackException and TrackTrace.
I use TrackEvent to log out things like start and end of methods of if something specific occurs that I want to log; TrackException is for logging Exception details and TrackTrace is for everything else.
telemetry.TrackEvent("Some Important Work Started");
try
{
telemetry.TrackTrace("I'm logging out the details of the work that is being done", SeverityLevel.Information);
}
catch(Exception ex)
{
telemetry.TrackException(ex);
}
telemetry.TrackEvent("Some Important Work Completed");
You now have the basics for logging. This will be useful to some extent, but it will be difficult to follow the traces when you have a busy system with logs of concurrent calls to the same methods. To assist you to filter your logs it would be useful to provide some identifying information that you can add to your logs to allow you to track and trace calls through your system. You could add these directly to your logs but this then makes your logs bloated and difficult to read. Application Insights provides a mechanism to pass properties along with the logs which will appear in the Log Analytics data that is returned from your query. Along with each log you can pass a dictionary of properties. I add to the set of properties as the code progresses to provide identifying information to assist with filtering the logs.I generally add in each new identifier as they are created. I can then use these in my queries to track the calls through my system and remove the ones I am not interested in. Diagnosing faults then becomes a lot easier. To make this work then you need to be consistent with the naming of the properties so that you always use the same name for the same property in different parts of the system. Also try and be consistent about when you use TrackEvent and TrackTrace. You can set levels for your traces based upon the severity level (Verbose, Information, Warning, Error, Critical)
TelemetryConfiguration.Active.InstrumentationKey = Key;
TelemetryClient telemetry = new TelemetryClient();
var logProperties = new Dictionary();
logProperties.Add("CustomerID", "the customer id pass through from elsewhere");
telemetry.TrackEvent("Some Important Work Started", logProperties);
try
{
var orderId = GenerateOrder();
logProperties.Add("OrderID", orderId.ToString());
telemetry.TrackTrace("I just created an order", logProperties);
var invoiceId = GenerateInvoice();
logProperties.Add("InvoiceID", invoiceId.ToString());
telemetry.TrackTrace("I've just created an invoice", logProperties);
SendInvoice(invoiceId);
}
catch (Exception ex)
{
telemetry.TrackException(ex, logProperties);
}
telemetry.TrackEvent("Some Important Work Completed", logProperties);
telemetry.Flush();
Flush needs to be called at the end to ensure that the data is sent to Log Analytics. In the code above you can see that I’ve added a CustomerId, OrderId and InvoiceId to the log properties and pass the log properties to each of the telemetry calls. Each of the logs will contain the properties that were set at the time of logging. I’ve generally wrap all this code so that I do not have to pass in the log properties into each call. I can add to the log properties whenever I have new properties and then each of the telemetry calls will include the log properties.
When we look at the logs via log analytics will can see the additional properties on the logs and then use them in our queries.
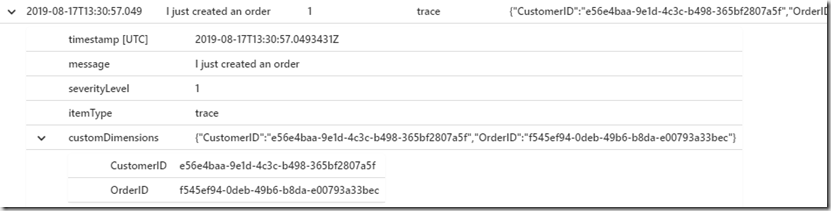
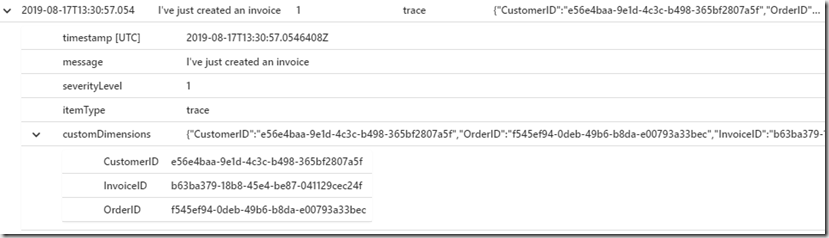
The log properties appear in customDimensions and you can see how the invoice log has the invoice id as well as the customer id and order id. The order log only has the customer id and order id.
You can add the custom dimensions to your queries as follows:
union traces, customEvents, exceptions
|order by timestamp asc
| where customDimensions.CustomerID == "e56e4baa-9e1d-4c3c-b498-365bf2807a5f"
You can also see in the logs the severity level which allows you to filter your logs to a sensible level. You need to plan your logs carefully and set an appropriate level to stop you flooding your logs with unnecessary data until you need it.
I’ve now shown you how to add logs to your application. You can find out more about the other methods available on the telemetry api here