In an earlier post I talked about using Graph API to invite users using B2B and add them to groups. In this post I am introducing the Beta version of the API. This has additional functionality that is currently not released. Microsoft have provided documentation for the Graph API which currently defaults to V1.0. See https://docs.microsoft.com/en-us/graph/api/overview
To see the Beta documentation there is a version drop down list which allows you to select either V1.0 or the Beta version.
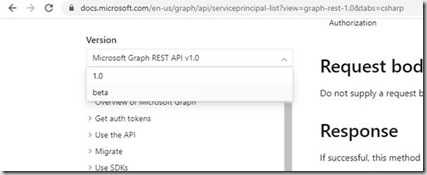
Just like the release version of Graph API you install the C# Graph API Beta using a nuget package: Microsoft.Graph.Beta
The code samples in here will work in both the Beta and released version but I wanted to show the difference between using the Beta API but also show you something you can use in production.
The scenario I am going to show is Adding a user to an Azure AD application.
First you will need a client to access the Beta Graph API. With the Beta API nuget package installed this automatically uses the Beta client.
ConfidentialClientApplicationOptions _applicationOptions = new ConfidentialClientApplicationOptions
{
ClientId = ConfigurationManager.AppSettings["ClientId"],
TenantId = ConfigurationManager.AppSettings["TenantId"],
ClientSecret = ConfigurationManager.AppSettings["AppSecret"]
};
var confidentialClientApp = ConfidentialClientApplicationBuilder
.CreateWithApplicationOptions(_applicationOptions)
.Build();
ClientCredentialProvider authProvider = new ClientCredentialProvider(confidentialClientApp);
GraphServiceClient betaGraphClient = new GraphServiceClient(authProvider);
This creates a Beta graph client with application scope.
To add an application role assignment to a user we need access to the Service Principles endpoint. Looking at the API documentation for List Service Principles we need an Application permission of Directory.ReadAll,
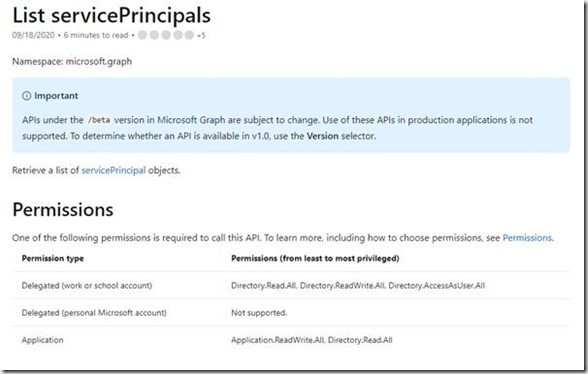
Create and Update Service Principles requires Directory.ReadWriteAll
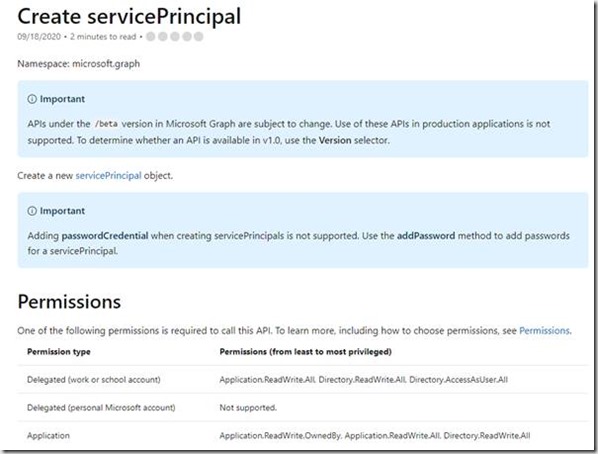
We can to add the permission to the Graph API application of Directory.ReadWriteAll and Grant Admin consent to the permissions we set up in the previous post. This will also cover the List API call.
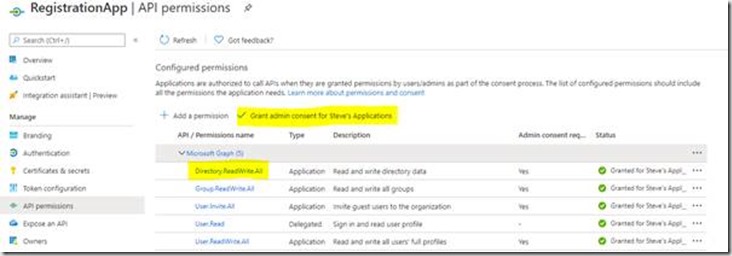
Without these permissions adding any calls to the Service Principle endpoint would return unauthorised.
In order to add an application role assignment we need to first obtain the user:
var user = (await betaGraphClient.Users.Request(options)
.Filter($"mail eq '{testUserEmail}'")
.GetAsync()).FirstOrDefault();
Then find all the app role assignments for the application we are interested in to see if the user is already assigned. To get the role assignments we call the service principle endpoint and expand the approleassignedto property:
var app1ServicePrincipals = await betaGraphClient.ServicePrincipals
.Request()
.Filter($"appId eq '{testApp1}'")
.Expand("approleassignedto")
.GetAsync();
var app1RoleAssignment = (from ra in app1ServicePrincipals[0].AppRoleAssignedTo
where ra.PrincipalId.ToString() == user.Id
select ra).FirstOrDefault();
If we want to remove the role assignment then we call the DeleteAsync method.
if (app1RoleAssignment != null)
{
await betaGraphClient.ServicePrincipals[app1ServicePrincipals[0].Id]
.AppRoleAssignedTo[app1RoleAssignment.Id]
.Request()
.DeleteAsync();
}
To Add a role assignment to the application call the Add endpoint:
app1RoleAssignment = new AppRoleAssignment
{
CreationTimestamp = DateTimeOffset.Now,
PrincipalDisplayName = user.DisplayName,
PrincipalId = Guid.Parse(user.Id),
PrincipalType = user.UserType,
ResourceDisplayName = app1ServicePrincipals[0].DisplayName,
ResourceId = Guid.Parse(app1ServicePrincipals[0].Id),
AppRoleId = Guid.Empty
};
await betaGraphClient.ServicePrincipals[app1ServicePrincipals[0].Id]
.AppRoleAssignments
.Request()
.AddAsync(app1RoleAssignment);
To see the role assignments that the user now has find all the appRoleAssignments that contain the users id:
var roleAssignments = servicePrincipals.SelectMany(x => x.AppRoleAssignedTo).ToList();
var appRoleAssignments = from ra in roleAssignments
where ra.PrincipalId.ToString() == user.Id
select ra;
foreach (var ra in appRoleAssignments)
{
Console.WriteLine($"[{ra.PrincipalDisplayName}] [{ra.ResourceDisplayName}]");
}
This should show the same information you can see when you look in Azure AD at the User’s applications:

Features and tools may change between Beta and Production. The code above changed slightly when moving to productions but the easiest way to see is to remove the Beta nuget and add the production one. The only change I had to make was to change the CreationTimeStamp to CreatedDateTime = DateTime.Now.
Information about which features are in Beta and which are in production can be found here: https://docs.microsoft.com/en-us/graph/whats-new-overview